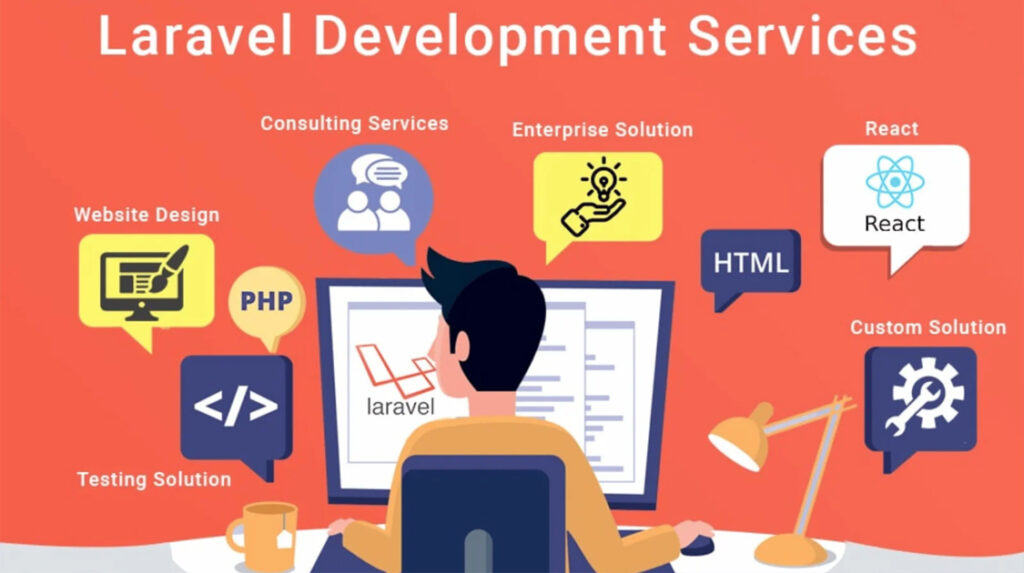
Laravel is a powerful PHP framework that allows for efficient and elegant web application development. It follows the Model-View-Controller (MVC) architectural pattern and provides a range of features and tools to streamline the development process.
Here’s an overview of Laravel development:
- Installation and Setup: Begin by installing Laravel on your local development environment or server. You can use Composer, a dependency management tool for PHP, to install Laravel and its dependencies. Follow the official Laravel documentation for detailed installation instructions.
- Project Configuration: Configure your Laravel project by setting up the database connection, application key, and other essential settings. Laravel provides a configuration file where you can define these settings based on your specific requirements.
- Routing: Define routes in Laravel to handle incoming HTTP requests and direct them to the appropriate controllers and actions. Routes can be defined in the routes/web.php file for web-based routes and routes/api.php for API routes. You can specify route parameters, middleware, and other settings to handle various types of requests.
- Controllers: Create controllers to handle the business logic of your application. Controllers receive requests from routes, process data, interact with models and services, and return responses. Use Laravel’s conventions and best practices to structure and organize your controllers effectively.
- Models: Define models to represent your application’s data and interact with the database. Laravel’s Eloquent ORM (Object-Relational Mapping) provides a convenient and expressive way to interact with databases. Define relationships between models and use Eloquent’s query builder to perform database operations.
- Views: Create views using Laravel’s Blade templating engine to define the presentation layer of your application. Blade provides a simple and powerful syntax for composing views and includes features like template inheritance, control structures, and reusable components. Pass data from controllers to views for dynamic rendering.
- Middleware: Utilize middleware in Laravel to add layers of functionality to your application’s HTTP requests. Middleware can perform tasks such as authentication, authorization, request modification, and response manipulation. Laravel provides several middleware out of the box and allows you to create custom middleware as needed.
- Authentication and Authorization: Implement user authentication and authorization in your Laravel application. Laravel’s built-in authentication scaffolding provides a complete system for user registration, login, and password reset. You can define authorization policies and gates to control access to specific parts of your application.
- Database Migrations and Seeders: Use Laravel’s migration system to manage database schema changes. Migrations allow you to version control and apply changes to the database structure. Seeders enable you to populate the database with test data or initial data required for your application.
- Testing: Write unit tests and integration tests to ensure the functionality and integrity of your Laravel application. Laravel provides a testing suite and utilities to facilitate automated testing. Test your controllers, models, routes, and other components to catch bugs and ensure the reliability of your application.
- Deployment: Prepare your Laravel application for deployment to a production environment. Optimize the application’s configuration, enable caching, and manage environment-specific settings. Configure a web server like Apache or Nginx to serve the application, and set up necessary permissions and security measures.
- Ongoing Maintenance and Support: Regularly update Laravel and its dependencies to benefit from bug fixes, security patches, and new features. Monitor your application’s performance, log and track errors, and address any issues that arise. Provide support and ongoing maintenance to ensure a smooth user experience.
Laravel’s extensive documentation and active community make it a popular choice for web application development. By leveraging Laravel’s features, tools, and best practices, you can build robust and scalable web applications efficiently.